Unreal: How how to subtract or add DateTime or Timespans
Implementing a little timer into my Unreal game I noticed there is some confusion as how to use DateTime and Timespan. Any calculation using two DateTime objects always results in a Timespan. DateTime is a full date, while Timespan a duration.
This is due to the C/C++ specification for DateTime and Timespan, the language Unreal Engine is written in. These specifications were created by experienced engineers, ergo Timespan is originally not intended to be calculated with. It’s possible there are C/C++ libraries made to deal with this problem.
However, you might want to also calculate via Timespans, to add to or subtract from a duration.
With some programming experience you can find a workaround by yourself.
High-Precision Solution
If you need to measure and calculate seconds and milliseconds, there is a solution using Timespans. We first break down both Timespans, do our calculations for each relevant field, then patch it back together using Make Timespan. It’s a bit clunky, but does the job.

The idea for this solution is the behavior of the “Make Timespan” node: it normalizes the parameters it receives, i.e. it clamps an input of 90 minutes on the minutes pin to 1 hour and 30 minutes, adding the hour remainder to the hour field, for example. Knowing this, we can simply perform our calculation per day/hour/etc.
I consider this a solution with the higher precision and performance. While it looks clunky, it is much easier to understand what it does.
The catch: it is best suited for smaller Timespans, as day is its largest unit. If you need months or years, you’ll have to do some additional conversions using Unreal.
Low-Precision Solution
Many programming languages (Java, C# and C/C++) have their date and time internally stored as a very large number (a long integer in some form), which is known as the Unix Time stamp, available in Java, C# and C/C++, among others. In Java you can obtain it by calling new Date().getTime(). The Unix Time stamp starts at midnight on 1st January 1970 and stores time passed ever since that date in seconds. It does not store milliseconds.
Now Unreal also provides a node for obtaining a Unix Time stamp. We can subtract or add dates by converting our DateTime objects into a Unix Time stamp, subtracting two very large numbers and then converting the result back into a DateTime object. In Unreal that’s a calculation with two 64-bit integers, so keep performance and memory usage in mind.
The below example is a little timer implemented using Unix Time stamp calculation.
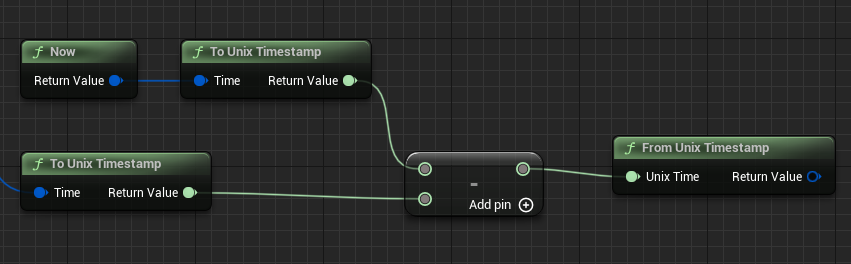
Also keep in mind with this solution we are bypassing valid and well-defined DateTime calculation operations. For example, the year, month, day and hour for above calculation will always be stuck at almost midnight on the 1st January 1970, if “Now” DateTime and “Time” DateTime are just a few seconds apart. This indicates the passed time for year, month and day corresponds to zero (with zero being 1st January 1970 at 00:00). But the measured difference in seconds is correct and can be used for further calculations. This solution works well for calculating large time differences.
The catch: While this solution is visually the simplest, the result must be interpreted and dealt with properly (e.g. you need to know what a Unix Time stamp is), which means bugs or errors can creep in easily. It is likely of poor performance (this is not something you should execute every frame) and might make problems during further calculations, so use this solution with caution.
If you want to display passed time in seconds or milliseconds on screen, you are better off by e.g. obtaining time delta from the engine and count it together every frame.
Hope this helps! Let me know which solution you settled with.